Composite Transformations on 2D
- theBloggers
- Mar 23, 2019
- 3 min read
Updated: Apr 2, 2019
Translation, Scaling, Rotation and Shearing a Car, made using graphics library in C.
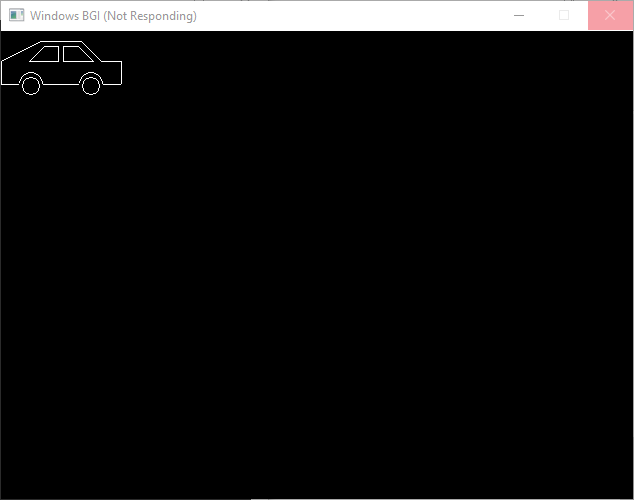
#include <graphics.h> /* include the necessary header files*/
#include <stdlib.h>
#include <stdio.h>
#include <conio.h>
#include <math.h>
void car(int, int);
void car(int, int, float);
void car(int, float, int);
int sx=1, sy=1;
int main(void)
{
int gd = DETECT, gm;
int i, maxx, midy, tx;
float ang, theta,shx;
/* initialize graphic mode */
initgraph(&gd, &gm,"");
/* maximum pixel in horizontal axis */
maxx = getmaxx();
/* mid pixel in vertical axis */
//midy = getmaxy()/2;
midy=30;
printf("\n\n 2D Transformations of Car: ");
car(0, midy);
printf("\n\n Enter the value of translation factor :"); // get the translation factor
scanf("%d",&tx);
delay(1);
printf("\n\n Next Operation is Scaling"); // get the scale factor
printf("\n\n Enter the Scale factor :");
scanf("%d %d",&sx,&sy);
car(tx,midy);
printf("\n\n Next Operation is Rotation");
printf("\n\n Enter the rotation angle :"); // get the angle of rotation
scanf("%f",&ang);
theta=((ang*3.14)/180); // convert the angle
car(130,130, theta);
printf("\nEnter the shear factor along x-axis:");
scanf("%f",&shx);
printf("\nXshear");
car(130,shx, 130);
getch();
closegraph();
return 0;
}
void car(int i, int midy)
{
int j=i;
for(i=0; i<=j; i++)
{
cleardevice();
line(i*sx, (midy + 23)*sy, i*sx, midy*sy);
line(i*sx, midy*sy, (40 + i)*sx, (midy - 20)*sy);
line((40 + i)*sx, (midy - 20)*sy, (80 + i)*sx, (midy - 20)*sy);
line((80 + i)*sx, (midy - 20)*sy, (100 + i)*sx, midy*sy);
line((100 + i)*sx, midy*sy, (120 + i)*sx, midy*sy);
line((120 + i)*sx, midy*sy, (120 + i)*sx, (midy + 23)*sy);
line((0 + i)*sx, (midy + 23)*sy,(18 + i)*sx, (midy + 23)*sy);
arc((30 + i)*sx, (midy + 23)*sy,0, 180, 12);
line((42 + i)*sx, (midy + 23)*sy, (78 + i)*sx, (midy + 23)*sy);
arc((90 + i)*sx, (midy + 23)*sy, 0, 180, 12);
line((102 + i)*sx, (midy + 23)*sy, (120 + i)*sx, (midy + 23)*sy);
line((28 + i)*sx, midy*sy, (43 + i)*sx, (midy - 15)*sy);
line((43 + i)*sx, (midy - 15)*sy, (57 + i)*sx, (midy - 15)*sy);
line((57 + i)*sx, (midy - 15)*sy, (57 + i)*sx, midy*sy);
line((57 + i)*sx, midy*sy, (28 + i)*sx, midy*sy);
line((62 + i)*sx, (midy - 15)*sy, (77 + i)*sx, (midy - 15)*sy);
line((77 + i)*sx, (midy - 15)*sy,(92 + i)*sx, midy*sy);
line((92 + i)*sx, midy*sy, (62 + i)*sx, midy*sy);
line((62 + i)*sx, midy*sy, (62 + i)*sx, (midy - 15)*sy);
circle((30 + i)*sx,(midy + 25)*sy, 9);
circle((90 + i)*sx, (midy + 25)*sy, 9);
delay(5);
}
}
void car(int i, int midy, float theta)
{
int j=i;
for(i=i; i<=j; i++)
{
cleardevice();
line(i*cos(theta)-(midy+23)*sin(theta), i*sin(theta)+(midy+23)*cos(theta), i*cos(theta)-midy*sin(theta), i*sin(theta)+midy*cos(theta));
line(i*cos(theta)-midy*sin(theta), i*sin(theta)+midy*cos(theta), (40 + i)*cos(theta)-(midy-20)*sin(theta),(40 + i)*sin(theta)+(midy-20)*cos(theta));
line((40 + i)*cos(theta)-(midy-20)*sin(theta), (40 + i)*sin(theta)+(midy-20)*cos(theta), (80 + i)*cos(theta)-(midy-20)*sin(theta), (80 + i)*sin(theta)+(midy-20)*cos(theta));
line((80 + i)*cos(theta)-(midy-20)*sin(theta), (80 + i)*sin(theta)+(midy-20)*cos(theta),(100 + i)*cos(theta)-(midy)*sin(theta), (100 + i)*sin(theta)+(midy)*cos(theta));
line((100 + i)*cos(theta)-(midy)*sin(theta), (100 + i)*sin(theta)+(midy)*cos(theta),(120 + i)*cos(theta)-(midy)*sin(theta), (120 + i)*sin(theta)+(midy)*cos(theta));
line((120 + i)*cos(theta)-(midy)*sin(theta), (120 + i)*sin(theta)+(midy)*cos(theta),(120 + i)*cos(theta)-(midy+23)*sin(theta), (120 + i)*sin(theta)+(midy+23)*cos(theta));
line((0 + i)*cos(theta)-(midy+23)*sin(theta), (0 + i)*sin(theta)+(midy+23)*cos(theta),(18 + i)*cos(theta)-(midy+23)*sin(theta), (18 + i)*sin(theta)+(midy+23)*cos(theta));
arc((30 + i)*cos(theta)-(midy+23)*sin(theta), (30 + i)*sin(theta)+(midy+23)*cos(theta), 0, 180, 12);
line((42 + i)*cos(theta)-(midy+23)*sin(theta), (42 + i)*sin(theta)+(midy+23)*cos(theta),(78 + i)*cos(theta)-(midy+23)*sin(theta), (78 + i)*sin(theta)+(midy+23)*cos(theta));
arc((90 + i)*cos(theta)-(midy+23)*sin(theta), (90 + i)*sin(theta)+(midy+23)*cos(theta), 0, 180, 12);
line((102 + i)*cos(theta)-(midy+23)*sin(theta), (102 + i)*sin(theta)+(midy+23)*cos(theta),(120 + i)*cos(theta)-(midy+23)*sin(theta), (120 + i)*sin(theta)+(midy+23)*cos(theta));
line((28 + i)*cos(theta)-(midy)*sin(theta), (28 + i)*sin(theta)+(midy)*cos(theta),(43 + i)*cos(theta)-(midy-15)*sin(theta), (43 + i)*sin(theta)+(midy-15)*cos(theta));
line((43 + i)*cos(theta)-(midy-15)*sin(theta), (43 + i)*sin(theta)+(midy-15)*cos(theta),(57 + i)*cos(theta)-(midy-15)*sin(theta), (57 + i)*sin(theta)+(midy-15)*cos(theta));
line((57 + i)*cos(theta)-(midy-15)*sin(theta), (57 + i)*sin(theta)+(midy-15)*cos(theta),(57 + i)*cos(theta)-(midy)*sin(theta), (57 + i)*sin(theta)+(midy)*cos(theta)); line((57 + i)*cos(theta)-(midy)*sin(theta), (57 + i)*sin(theta)+(midy)*cos(theta),(28 + i)*cos(theta)-(midy)*sin(theta), (28 + i)*sin(theta)+(midy)*cos(theta));
line((62 + i)*cos(theta)-(midy-15)*sin(theta), (62 + i)*sin(theta)+(midy-15)*cos(theta),(77 + i)*cos(theta)-(midy-15)*sin(theta), (77 + i)*sin(theta)+(midy-15)*cos(theta));
line((77 + i)*cos(theta)-(midy-15)*sin(theta), (77 + i)*sin(theta)+(midy-15)*cos(theta),(92 + i)*cos(theta)-(midy)*sin(theta), (92 + i)*sin(theta)+(midy)*cos(theta));
line((92 + i)*cos(theta)-(midy)*sin(theta), (92 + i)*sin(theta)+(midy)*cos(theta),(62 + i)*cos(theta)-(midy)*sin(theta), (62 + i)*sin(theta)+(midy)*cos(theta));
line((62 + i)*cos(theta)-(midy)*sin(theta), (62 + i)*sin(theta)+(midy)*cos(theta),(62+ i)*cos(theta)-(midy-15)*sin(theta), (62 + i)*sin(theta)+(midy-15)*cos(theta));
circle((30 + i)*cos(theta)-(midy+25)*sin(theta), (30+i)*sin(theta)+(midy + 25)*cos(theta), 9);
circle((90 + i)*cos(theta)-(midy+25)*sin(theta), (90+i)*sin(theta)+(midy + 25)*cos(theta), 9);
delay(50);
}
}
void car(int i, float shx, int midy)
{
int j=i;
for(; i<=j; i++)
{
cleardevice();
line(i+(midy+23)*shx, (midy + 23), i+(midy*shx), midy);
line(i+(midy)*shx, midy, (40+i)+((midy-20)*shx), midy-20);
line(40+i+(midy-20)*shx, (midy -20), 80+i+((midy-20)*shx), midy-20);
line(80+i+(midy-20)*shx, (midy - 20), 100+i+(midy*shx), midy);
line(100+i+(midy*shx), midy , 120+i+(midy*shx), midy);
line(120+i+(midy*shx), midy, 120+i+((midy+23)*shx), midy+23);
line(i+(midy+23)*shx, (midy + 23), 18+i+((midy+23)*shx), midy+23);
arc((30 + i)+(midy+23)*shx, (midy + 23),0, 180, 12);
line(42+i+((midy+23)*shx), (midy + 23), 78+i+((midy+23)*shx), midy+23);
arc((90 + i)+((midy+23)*shx), (midy + 23), 0, 180, 12);
line(102+i+((midy+23)*shx),midy + 23, 120+i+((midy+23)*shx), midy+23);
line(28+i+(midy*shx), midy, 43+i+((midy-15)*shx), midy-15);
line(43+i+((midy-15)*shx), (midy -15), 57+i+((midy-15)*shx), midy-15);
line(57+i+((midy-15)*shx), (midy -15), 57+i+(midy*shx), midy);
line(57+i+(midy*shx), midy, 28+i+(midy*shx), midy);
line(62+i+((midy-15)*shx), (midy -15), 77+i+((midy-15)*shx), midy-15);
line(77+i+((midy-15)*shx), (midy -15), 92+i+(midy*shx), midy);
line(92+i+(midy*shx), midy, 62+i+(midy*shx), midy);
line(62+i+(midy*shx), midy, 62+i+((midy-15)*shx), midy-15);
circle((30 + i)+((midy + 25)*shx),midy+25, 9);
circle((90 + i)+((midy + 25)*shx),midy+25, 9);
delay(5);
}
}


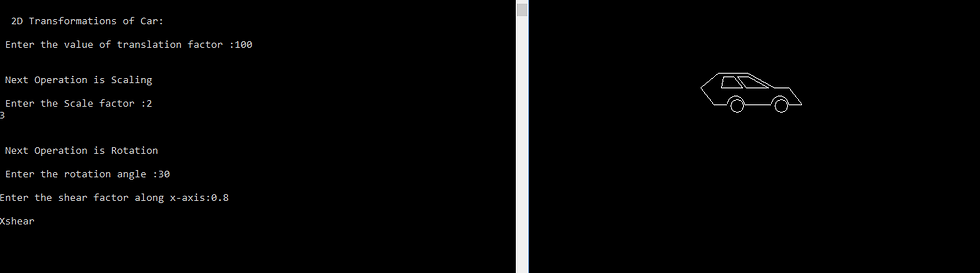
コメント