DIGITAL ASSIGNMENT 2: Panoramic View Projections
- theBloggers
- Mar 29, 2019
- 1 min read
Updated: Apr 1, 2019
Name: Bhavya S Chauhan
Reg. No. :17BCE1270
Youtube link: https://youtu.be/c6kfOq1nOws
Name: Ibra Nafis
Reg. No. :17BCE1230
Youtube link: https://youtu.be/1HrK3mxlcDg
Panoramic images create a photo-realistic, 3 dimensional, navigable environment, by capturing the surrounding of the image in a 360 degree cylindrical or spherical view.
Panoramic images are implemented extensively in adventure style games with immersive experience and in real estate images to give guided tours of museums and building sites.
Here I have discussed the basic algorithm for panoramic image projection and shown the implementation of a program to stitch images together by using mathematical model to map pixel coordinates from one image to another.
Program (Python and OpenCV):
# USAGE
# python image_stitching_simple.py --images scottsdale --output output.png
# import the necessary packages
from imutils import paths
import numpy as np
import argparse
import imutils
import cv2
# construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--images", type=str, required=True,
help="path to input directory of images to stitch")
ap.add_argument("-o", "--output", type=str, required=True,
help="path to the output image")
args = vars(ap.parse_args())
# grab the paths to the input images and initialize our images list
print("[INFO] loading images...")
imagePaths = sorted(list(paths.list_images(args["images"])))
images = []
# loop over the image paths, load each one, and add them to our
# images to stitch list
for imagePath in imagePaths:
image = cv2.imread(imagePath)
images.append(image)
# initialize OpenCV's image sticher object and then perform the image
# stitching
print("[INFO] stitching images...")
stitcher = cv2.createStitcher() if imutils.is_cv3() else cv2.Stitcher_create()
(status, stitched) = stitcher.stitch(images)
# if the status is '0', then OpenCV successfully performed image
# stitching
if status == 0:
# write the output stitched image to disk
cv2.imwrite(args["output"], stitched)
# display the output stitched image to our screen
cv2.imshow("Stitched", stitched)
cv2.waitKey(0)
# otherwise the stitching failed, likely due to not enough keypoints)
# being detected
else:
print("[INFO] image stitching failed ({})".format(status))
Output:
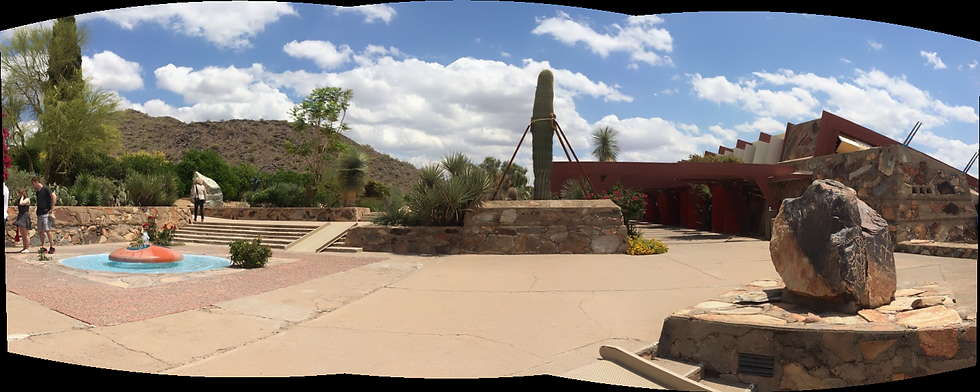
Comments